When building a web application with Next.js, handling email functionality is a crucial requirement for features like contact forms, notifications, and transactional emails. But what’s the best way to send emails in Next.js, especially when hosted on serverless platforms like Vercel? Here’s a comprehensive guide to tools, third-party services, and best practices for email-sending in Next.js applications.
Why Emails Matter in Next.js
Emails are essential for:
- Contact Forms: Allowing users to reach out.
- Transactional Emails: Sending confirmations, password resets, and receipts.
- Notifications: Keeping users and admins informed.
Next.js, with its serverless architecture, makes it easy to implement email functionality via API routes. Here’s how.
Native Solution: Using Nodemailer
Nodemailer is a popular Node.js library for sending emails via SMTP. It integrates well with Next.js API routes, offering a flexible, self-hosted solution.
Setting Up Nodemailer in Next.js
- Install Nodemailer:
npm install nodemailer
- Create an API Route: Create a file like
/app/api/contact/route.ts
to handle the email logic using Next.js 15 and TypeScript. - Configure SMTP:
import nodemailer from 'nodemailer';
import { NextResponse } from 'next/server';
export async function POST(request: Request) {
const body = await request.json();
const { email, message } = body;
const transporter = nodemailer.createTransport({
service: 'Gmail', // Or use another SMTP provider
auth: {
user: process.env.SMTP_USER,
pass: process.env.SMTP_PASS,
},
});
try {
await transporter.sendMail({
from: process.env.SMTP_USER,
to: 'admin@example.com',
subject: 'New Contact Form Submission',
text: `Message from ${email}: ${message}`,
});
return NextResponse.json({ success: true });
} catch (error) {
console.error(error);
return NextResponse.json({ success: false });
}
}
Pros:
- Complete control over the email process.
- Works with any SMTP server (Gmail, Outlook, etc.).
Cons:
- Requires managing SMTP credentials securely.
- Deliverability depends on your SMTP provider.
Third-Party Email Services
Third-party services make email handling easier, especially in serverless environments. Here are the most popular options:
1. SendGrid
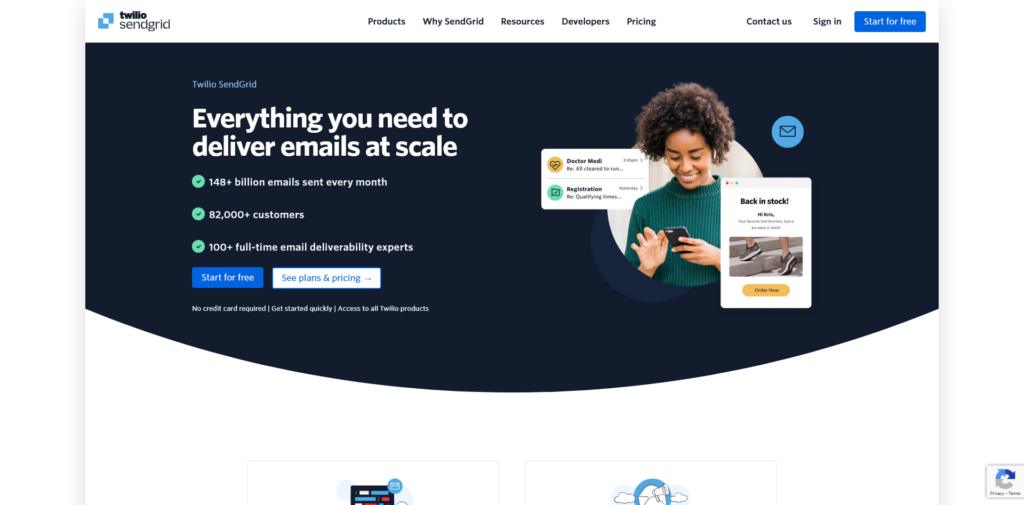
SendGrid is a cloud-based email service known for its reliability and scalability.
- Free Tier: 100 emails/day.
- Features: Transactional emails, email tracking, templates.
- Why Use It: Integration-friendly, ideal for serverless platforms like Vercel.
- Fit For: Hobbyists and professionals.
- Stat: SendGrid powers over 58,000 businesses globally.
2. Mailgun
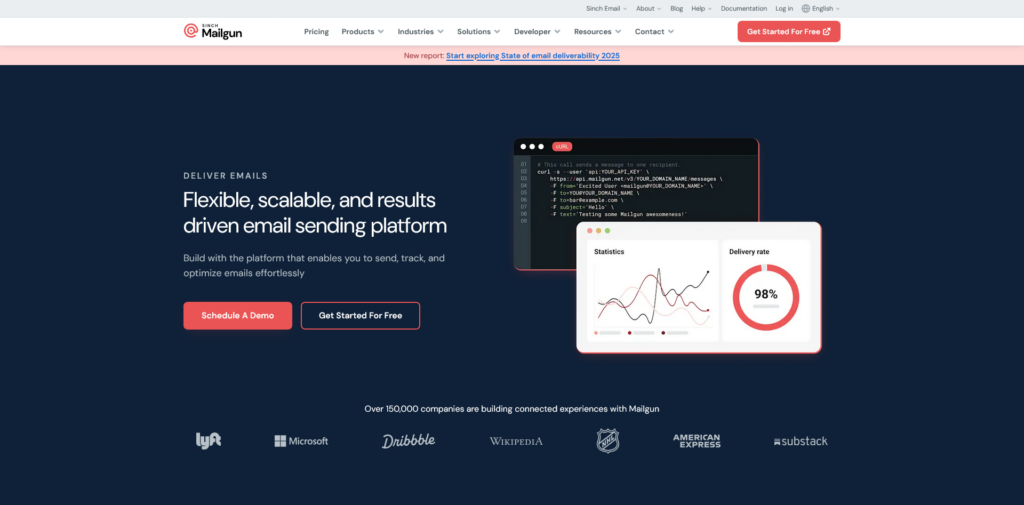
Mailgun specializes in transactional emails and analytics.
- Free Tier: 5,000 emails/month (first month only).
- Features: Advanced analytics, deliverability monitoring.
- Why Use It: High deliverability rates, RESTful API.
- Fit For: Professionals.
- Stat: Used by 150,000 businesses worldwide.
3. Postmark
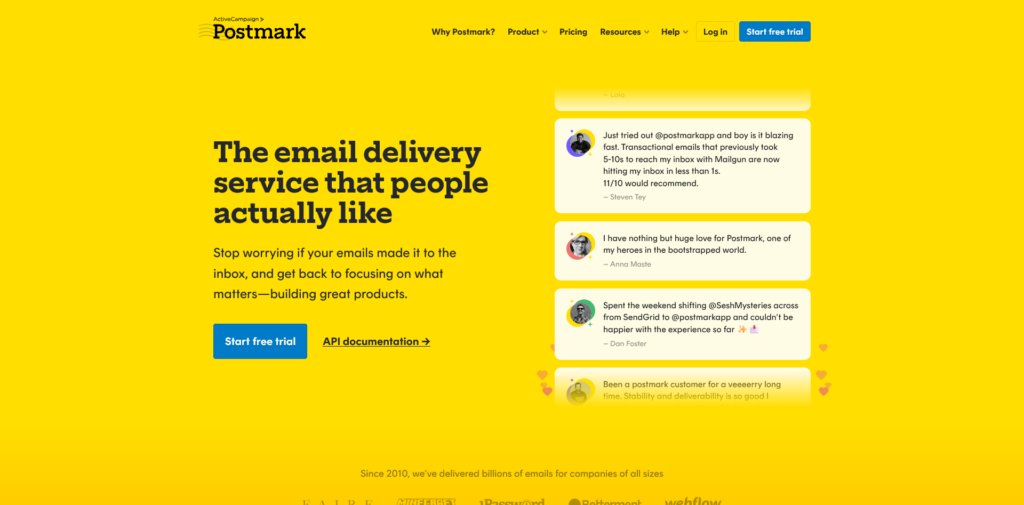
Postmark is designed specifically for transactional emails.
- Free Tier: 100 emails/month.
- Features: Fast delivery, email tracking.
- Why Use It: Focuses solely on transactional emails.
- Fit For: Hobbyists and professionals.
- Stat: 97% of emails are delivered in under 1 second.
4. Amazon SES
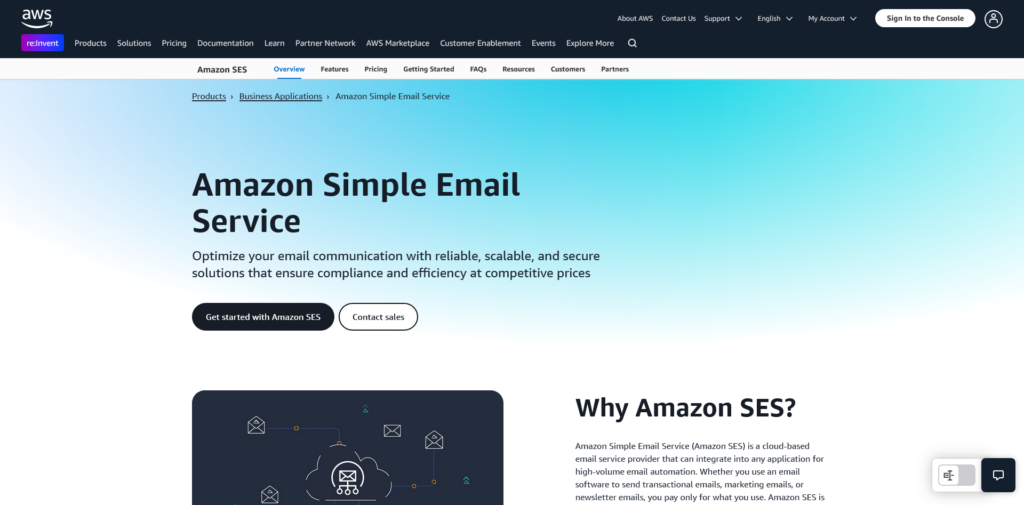
Amazon Simple Email Service (SES) is a cost-effective solution for high-volume email needs.
- Pricing: $0.10 per 1,000 emails.
- Features: Scalable, integrates with other AWS services.
- Why Use It: Perfect for high-volume email systems.
- Fit For: Professionals.
- Stat: AWS dominates 32% of the cloud infrastructure market.
5. EmailJS
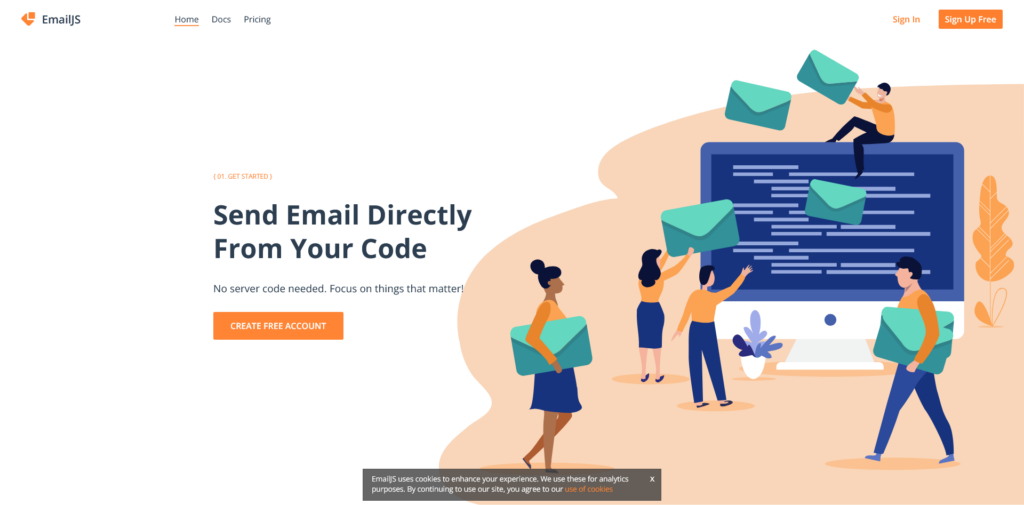
EmailJS allows you to send emails directly from the client side without a backend.
- Free Tier: Limited templates.
- Features: Client-side email sending.
- Why Use It: Great for simple setups, but security concerns exist.
- Fit For: Personal use and hobbyists.
6. Resend
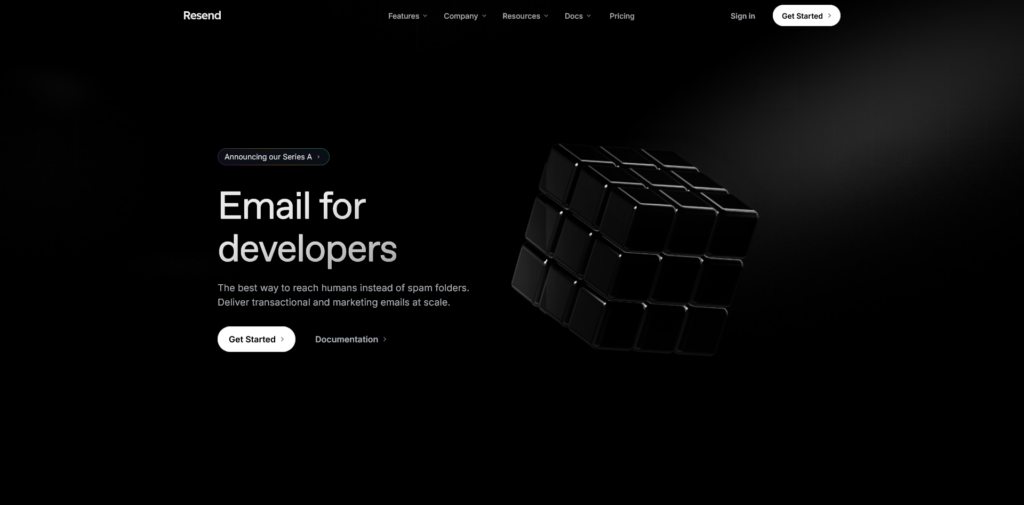
Resend is a modern email-sending service designed for developers.
- Pricing: $0.004 per email.
- Features: Developer-friendly APIs, focus on transactional emails.
- Why Use It: Simple, fast integration.
- Fit For: Hobbyists and professionals.
7. Tally.so (via Integrations)
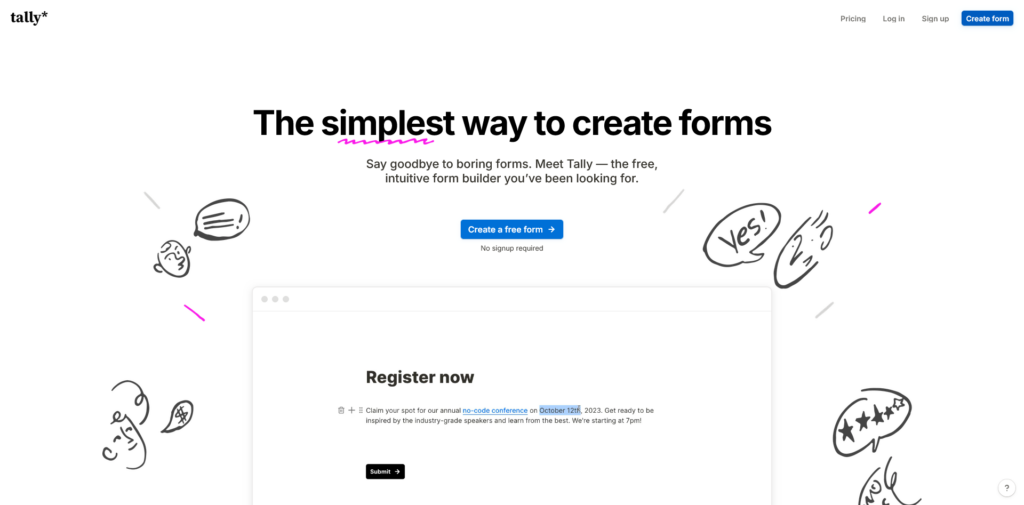
Tally.so is primarily a form builder but integrates with email services like Zapier for email workflows.
- Free Tier: Form building only.
- Features: Collect form submissions and trigger emails.
- Why Use It: Combine form-building and email workflows seamlessly.
- Fit For: Hobbyists.
Comparison Table
Service | Free Tier | Starting Price | Features | Fit For |
---|---|---|---|---|
Nodemailer | N/A | Self-hosted | Full SMTP control | Hobbyists and professionals |
SendGrid | 100 emails/day | $15/month | Templates, tracking | Hobbyists and professionals |
Mailgun | 5,000 emails (first month) | $35/month | Deliverability monitoring | Professionals |
Postmark | 100 emails/month | $10/month | Fast transactional emails | Hobbyists and professionals |
Amazon SES | Pay-as-you-go ($0.10 per 1,000 emails) | Variable | High-volume scalability | Professionals |
EmailJS | Free for limited use | Variable | Client-side email sending | Personal use and hobbyists |
Resend | N/A | $0.004/email | Developer-friendly APIs | Hobbyists and professionals |
Tally.so | Free (form building only) | Variable | Integrates with Zapier, others | Hobbyists |
Choosing the Right Option
- For Full Control: Use Nodemailer if you prefer managing your own SMTP server.
- For Serverless Environments: SendGrid, Mailgun, or Postmark offer easy integrations with platforms like Vercel.
- For High Volume: Amazon SES is cost-effective for large-scale email needs.
- For Simplicity: EmailJS or Resend is ideal for quick setups and lightweight use cases.
- For Analytics: SparkPost offers excellent tracking and insights.
Best Practices for Email Integration in Next.js
- Secure Your Credentials: Store API keys and SMTP credentials in environment variables.
- Validate User Input: Prevent spam and abuse by validating form submissions.
- Monitor Deliverability: Use tools like SendGrid’s dashboard or SparkPost’s analytics to track performance.
- Test Before Deploying: Always test your email logic locally and in staging environments.
Conclusion
Sending emails in Next.js doesn’t have to be complicated. Whether you choose a self-hosted solution like Nodemailer or a third-party service like SendGrid or Postmark, the right approach depends on your project’s needs. By leveraging these tools and following best practices, you can implement a reliable email system that enhances user experience and keeps your app running smoothly.
So, what’s your pick? Nodemailer for control, or a service like SendGrid for simplicity? Either way, you’re just a few lines of code away from seamless email integration!